Hide / Show Panel on client side with button click using java script
<head runat="server">
<title>show hide on client side</title>
<script language="javascript" type="text/javascript">
function click_button()
{
if(document.getElementById('<%=Panel1.ClientID%>').style.display == 'none')
{
document.getElementById('<%=Panel1.ClientID%>').style.display = 'block';
document.getElementById('btnshowHide').value = 'hide';
}
else
{
document.getElementById('<%=Panel1.ClientID%>').style.display = 'none';
document.getElementById('btnshowHide').value = 'show';
}
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<input id="btnshowHide" type="button" value="hide" onclick="click_button();" />
<asp:Panel ID="Panel1" runat="server" Height="50px" Width="125px">
click hide to hide this text
</asp:Panel>
</div>
</form>
</body>
To enable client side click we are using a html button
<input id="btnshowHide" type="button" value="show" onclick="click_button();" />
In the method click_button() we are accessing the panel to hide using the client id of the control
document.getElementById('<%=Panel1.ClientID%>').style.display
so now to hide we set the style as 'none'
document.getElementById('<%=Panel1.ClientID%>').style.display = 'none';
and to show it back we set style as 'block'
document.getElementById('<%=Panel1.ClientID%>').style.display = 'block';
Also we toggle the text of the button between show and hide
document.getElementById('btnshowHide').value = 'show';
image when intially loaded
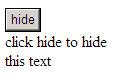
when hide is clicked, this is how shown, every thing done on client side

Madhu :-)
Labels: acessing panel, asp.net, asp.net javascript, javascript, show hide panel